Autoprefixer
Saturday, June 4th, 2016 | Programming
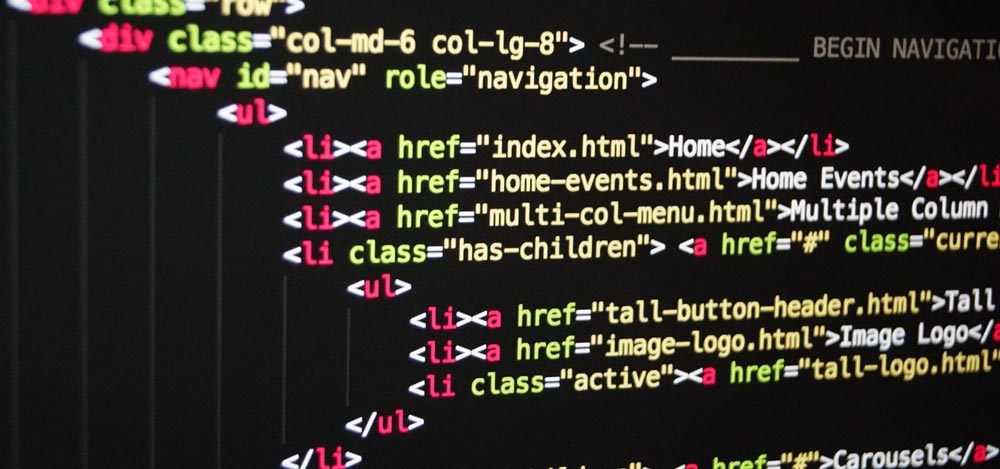
Web browsers come in various shapes and sizes: different users will have different ones, and inevitably different versions of the same one. When CSS3 arrived browsers began adding support for it before the specification had been finalised and so used vendor prefixes.
The result, now we have a standardised CSS3, is that some users have proper CSS3 support and some have the support, but only behind a complicated series of vendor names. Therefore, if you want to use flexbox for example, you cannot just rely on display: fiex;
as for some users it will only work with the appropriate vendor prefix.
This means you have to tediously insert all of these vendor prefix statements to get cross-browser compatibility. However, there is a tool called Autoprefixer that takes this hassle away. It is an NPM module that converts your regular CSS into CSS with vendor prefixes. You write:
display: flex;
And you get:
display: -webkit-flex;
display: -ms-flexbox;
display: flex;
This means you have to compile your CSS. However, if you are already compiling from LESS or SASS, it’s really easy to integrate it. On one of my current projects I already had Gulp compiling SASS, so it was one-liner to add the step in. SitePoint have a tutorial on how to set it up.
Web browsers come in various shapes and sizes: different users will have different ones, and inevitably different versions of the same one. When CSS3 arrived browsers began adding support for it before the specification had been finalised and so used vendor prefixes.
The result, now we have a standardised CSS3, is that some users have proper CSS3 support and some have the support, but only behind a complicated series of vendor names. Therefore, if you want to use flexbox for example, you cannot just rely on display: fiex;
as for some users it will only work with the appropriate vendor prefix.
This means you have to tediously insert all of these vendor prefix statements to get cross-browser compatibility. However, there is a tool called Autoprefixer that takes this hassle away. It is an NPM module that converts your regular CSS into CSS with vendor prefixes. You write:
display: flex;
And you get:
display: -webkit-flex; display: -ms-flexbox; display: flex;
This means you have to compile your CSS. However, if you are already compiling from LESS or SASS, it’s really easy to integrate it. On one of my current projects I already had Gulp compiling SASS, so it was one-liner to add the step in. SitePoint have a tutorial on how to set it up.