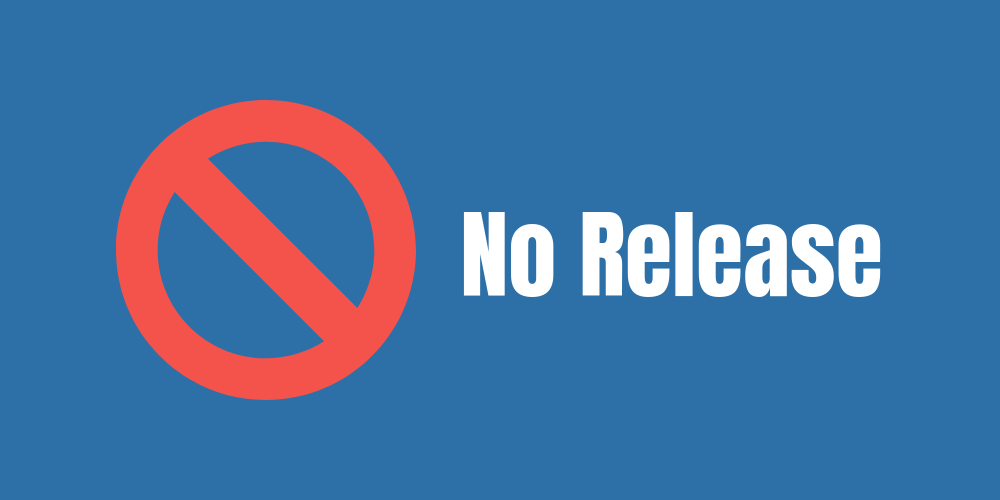
You’ve no doubt heard of us at Glorry, the exciting Silicon Valley startup that is taking the world by storm. We’re best known for raising £17 billion in funding on Kickstart in less than 38 minutes, despite having no discernable business model. Still, that’s what they said about Instagram and look who is laughing now. Mark Zuckerberg, that’s who.
We’re pushing the limits of Agile delivery to see how we can deliver the most value to our customers. But our Service Delivery team are also looking to ensure a consistent and stable customer experience that doesn’t allow new features to compromise on quality.
The result is a methodology we’ve called “No-Release” and I’m excited to share some details of it with you today.
What is No-Release?
Simply put, we don’t release any code.
What are the results like?
They’ve been outstanding. Since we adopted this approach, we’ve had zero bugs introduced to the live system. That’s not a misprint: zero bugs. Not one single incident has been released related to the new code we’ve been writing.
Since adopting this approach, our velocity has increased. Developers feel more confident that their work will not cause issues in live. Product Owners are happy to prioritise tech debt because they know it won’t delay new features arriving in live. Service Delivery is less jittery about degradation due to changes to the product.
How does it work?
We based our workflow on a traditional Scrum methodology. We operate in two-week sprints with a backlog of features prioritised by the Product Owner. Each ticket begins with a Business Analyst sitting down with a Developer and a Tester to work out how we can deliver and test the acceptance criteria of the ticket.
When a ticket is complete and signed off, including going through our continuous integration pipeline where a series of automated tests are run, we then merge the ticket into our develop branch. At this point, the ticket reaches our Definition of Done and we can close it.
Our master branch contains a copy of the code deployed to live, while our develop branch contains all of the new features. Because we operate under No-Release, we almost never have to deal with merge conflicts because we never merge develop into master. Or anything else for that matter.
What are the drawbacks?
One of the biggest drawbacks to No-Release is that you do not release any code. This means that no new features and improvements ever make it to the end user.
Making this work requires buy-in across the organisation. Without everyone being on board you can easily get developers saying “this is pointless, what am I doing here” every stand-up, and upper management suggesting they can fire the entire team and get the same results for much less money. Therefore, it’s important to get everything to embrace the methodology before starting.
Each organisation needs to make its own decision as to whether this drawback is acceptable to gain the benefits discussed above.
Conclusion
No-Release methodology allows you to increase your development velocity while eliminating any risk of service disruption to the end user.