Modernizing Legacy Applications In PHP
Tuesday, December 8th, 2015 | Books, Programming
Modernizing Legacy Applications In PHP is a book by Paul M. Jones on how to bring legacy codebases up to date. Jones is a relatively big-whig in the PHP community, being involved in most of the PSR standards and a contributor to the Zend Framework.
For me, the book was both excellent and uninformative. What I mean by that is that I do not think I learnt anything from reading it. However, I flatter myself that I am pretty good at PHP and have spent many years working with legacy codebases. It was always been an interest of mine, and I have previously submitted conference papers on the subject. I have been doing just what this book describes with my current client.
That in itself shows the quality of the book though. It describes every step I have been going through in a logical and clear order. It explains introducing autoloading, separating out the concerns, adding unit testing and injecting your dependencies. It says you from the tangled mess to a clean and modern application in an easy-to-follow manner. In short, it is pretty much everything I would recommend to someone starting to clean up a legacy application.
It has code examples and some tips and tricks in it, but for the most part it is quite high level. It also deals with PHP 5.0 and onwards. There is perhaps room to expand here. At Buzz I had to come up with techniques to support ancient problems like register globals, and at the NHS I ran into PHP 4.6 installed on their servers. Solving these kind of problems, and the little code tricks you can do, might have been a useful additional also.
Overall, I would recommend this book to anyone who feels the least bit daunted by the idea of modernising a legacy PHP codebase. It is clear, easy-to-follow and takes you through exactly the steps you should follow.
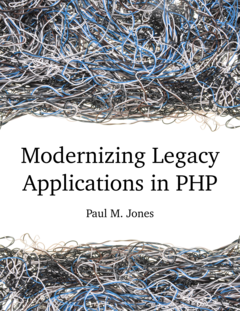
Modernizing Legacy Applications In PHP is a book by Paul M. Jones on how to bring legacy codebases up to date. Jones is a relatively big-whig in the PHP community, being involved in most of the PSR standards and a contributor to the Zend Framework.
For me, the book was both excellent and uninformative. What I mean by that is that I do not think I learnt anything from reading it. However, I flatter myself that I am pretty good at PHP and have spent many years working with legacy codebases. It was always been an interest of mine, and I have previously submitted conference papers on the subject. I have been doing just what this book describes with my current client.
That in itself shows the quality of the book though. It describes every step I have been going through in a logical and clear order. It explains introducing autoloading, separating out the concerns, adding unit testing and injecting your dependencies. It says you from the tangled mess to a clean and modern application in an easy-to-follow manner. In short, it is pretty much everything I would recommend to someone starting to clean up a legacy application.
It has code examples and some tips and tricks in it, but for the most part it is quite high level. It also deals with PHP 5.0 and onwards. There is perhaps room to expand here. At Buzz I had to come up with techniques to support ancient problems like register globals, and at the NHS I ran into PHP 4.6 installed on their servers. Solving these kind of problems, and the little code tricks you can do, might have been a useful additional also.
Overall, I would recommend this book to anyone who feels the least bit daunted by the idea of modernising a legacy PHP codebase. It is clear, easy-to-follow and takes you through exactly the steps you should follow.