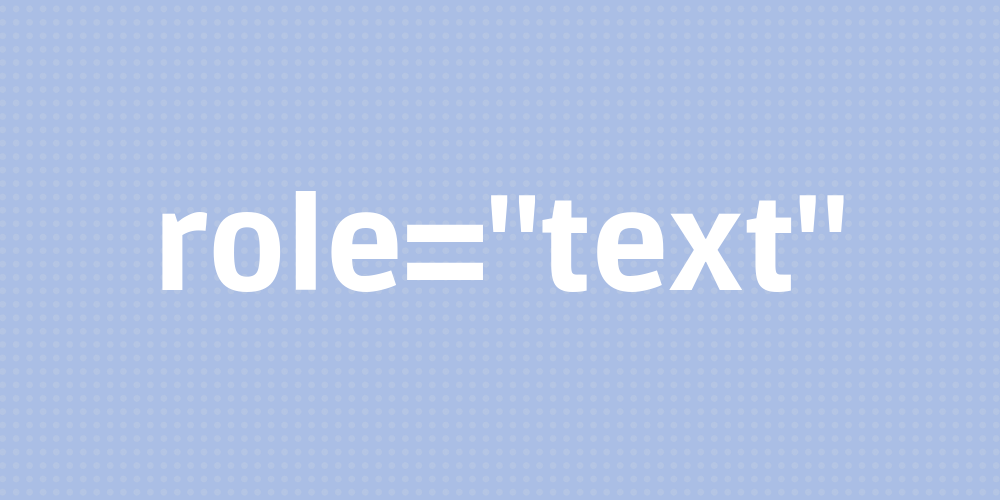
You’re trying to make your website accessible. Lovely stuff. But because screen reader technology is so bad, you need to add a bunch of inline span
tags with the aria-label
attribute on them so that you can add additional context, or, more usually, use some kind of hack to get around a screen reader bug.
This works well. However, it also splits the whole thing into separate groups. Consider the following example:
An annual subscription costs £20.00 per year.
Looks good. Except for VoiceOver on Mac and iOS, and probably other screen readers, too, will read out something like this:
Pound two zero zero zero
Oh no! It totally ignored the decimal point and now your user, if they are able to track the weird way it read out individual numbers, thinks that your product costs two thousand pounds. So, our old friend the Aria label is here to help.
An annual subscription costs <span aria-label=”twenty pounds”>£20.00</span> per year.
Hurray! It now sounds like normal language. But it’s still confusing. Now the screenreader reads them out as three separate blocks. “An annual subscription.” “Twenty pounds, group.” “Per year.” The user has to navigate through all three of them even though it is one sentence.
The fix
To fix it, we can use the role
attribute. Sometimes.
If we set it to role="text"
it will work in WebKit. For example:
<span role=”text”>An annual subscription costs <span aria-label=”twenty pounds”>£20.00</span> per year.</span>
Now it will read out the entire sentence as one string, but still using the Aria label.
Is role=”text” a thing?
No. That’s the drawback. it was proposed to be included in the Aria spec, but nobody could agree on whether it should be a thing or not. So, it was left out. Therefore, it is not implemented everywhere.
It is implemented in WebKit, so will fix the problem on Chrome, iOS, etc. But it won’t fix it in some other browsers. And, you might get pulled up by linting tools and validators because it is not part of any formal specification.
Where can I put it?
Anywhere you like given that it is a made up attribute.
But there are some things to be cautious of. It should only go on a block of text where you do not mind losing any of the context inside of it. If you have an element inside the paragraph, for example, that should be treated as a separate group, then leave it off.
It shouldn’t on headings because you don’t want to lose the context. Instead, put a span tag inside the heading with it on.
Also, you need to ensure you are using the aria-label
attribute for anything inside. Normally, you can use an abbr
tag with a title
attribute and the screen reader will read out the title. However, if you wrap it in a role="text"
, this will only work if you use an aria-label
instead/as well as the title attribute.